f
What is Django?#
Django is a high-level, web framework written in Python for rapid development and pragmatic design. A framework is simply a collection of modules that are grouped together for creating web applications from a reliable, pre-existing existing source. Django offers a large collection of theses modules.
Django was created in 2003 when web developers working for a newspaper company in Lawrence, Kansas, needed a better way to organize their code. Because the developers were surrounded by many newspaper journalists, clear documentation became an integral part of the project that became known as the “Django” project.
Django has since blossomed into a massive online open-source community that has a solution for just about everything you can think of, from authentication to content management systems.
Benefits of Django#
There are several benefits to using Django over other possible solutions:
- Fast: because of the way Django is set up, you can get off the ground very quickly. It doesn’t really take any time at all to get a Django application setup if you have the architecture of the app already in mind.
- Scalable: Django can meet the traffic demands of a large project.
- Fully loaded: there are all sorts of packages that you can use to carry out standard web application tasks like authentication or content administration or querying. It’s all pre-baked in.
- Versatile: Django is fairly versatile. You can use it for all sorts of applications. The sky is the limit really on what you can do with it.
- Secure: common security risks are averted with Django’s built-in security protocols for cross-site request forgeries, cross-site scripting, clickjacking, and SQL injection.
- SEO optimized: Django makes SEO easier by maintaining a website through URLs rather than IP addresses.
- Documentation: Django’s documentation is one of the best on the market. It’s easy to read, even for people with no technical background.
With Django’s versatility, it’s certainly capable of meeting your project’s needs. Companies like Spotify, Pinterest, National Geographic, and Dropbox use Django for their business models. Let’s take a look next at the common design patterns that these companies have used so you can get a feel for the shape of a typical project.
Understanding the Django Framework#
As highlighted earlier, Django — a high-level Python web framework, offers a robust, reliable, and efficient solution for building web applications and embraces a “batteries-included” concept by delivering many built-in and ready-to-use web development tools and components.
This course will dive deep into the core components of the Django framework and explore its various features. It will specifically teach you the following:
-
Creating routes (or views): Views in the Django framework define what is displayed when a specific URL is requested.
-
Serving static content and files: You will learn how to configure and serve static files such as stylesheets, images, and JavaScript using Django’s built-in tools.
-
Connect templates with models to serve data dynamically: You will explore how to connect templates (the presentation layer) with models (the data layer) to display a web page.
-
Content views: This section will cover the process of defining models and their relationships by binding them with templates and views to create a fully functional web application.
-
Working with Databases using SQLite: In this part of the course, you will learn how to work with SQLite and perform common CRUD (create, read, update, and delete) database operations.
-
Handle and validate forms: Forms are essential to web applications by allowing users to input and submit data. This section will teach you how to create, handle, and validate forms using Django’s form system.
-
Create relative URLs with templates and explore template and custom filters: You will be guided on how to use relative URLs in your templates, which will result in versatile and easy to maintain applications.
-
User models, forms, and authentication: Not only will you learn how to create user models, registrations, and login forms, but you will also learn to implement them using Django’s built-in authentication system.
-
Building a Django application practically: Throughout the course, you will apply each section’s concepts and techniques to create a fully-functional Django application. This hands-on approach will reinforce your understanding of Django’s features and give you the practical experience to confidently develop your future web applications.
Learning Django Programming#
Diving into Django with Python programming will open a vast world of web development opportunities for you. Because Django is a high-level Python web framework that is popular for its swift development, clean design, and scalability, it will simplify complex tasks and allow you to focus on writing efficient code.
The keys to learning Django includes:
- Understanding Python programming
- Acquainting yourself with Django’s components (models, views, templates, and forms)
- Working hands-on with real-world projects
Practical experience with small or big projects will help solidify your understanding of the framework.
Additionally, acquainting yourself with Django’s vast array of third-party packages, including the Django REST framework, Django-tenants, and Django-crispy-forms can streamline your development experience.
That being said, learning Django Python is an engaging and rewarding journey that will equip you with the skills needed to create dynamic and interactive web applications.
Django Design Pattern#
There are three major components to Django’s architecture: elements that aid in working with the database, a template system that works for people who don’t program, and a framework that automates a lot of the website management. This lends itself to a Model, View, Template design pattern:
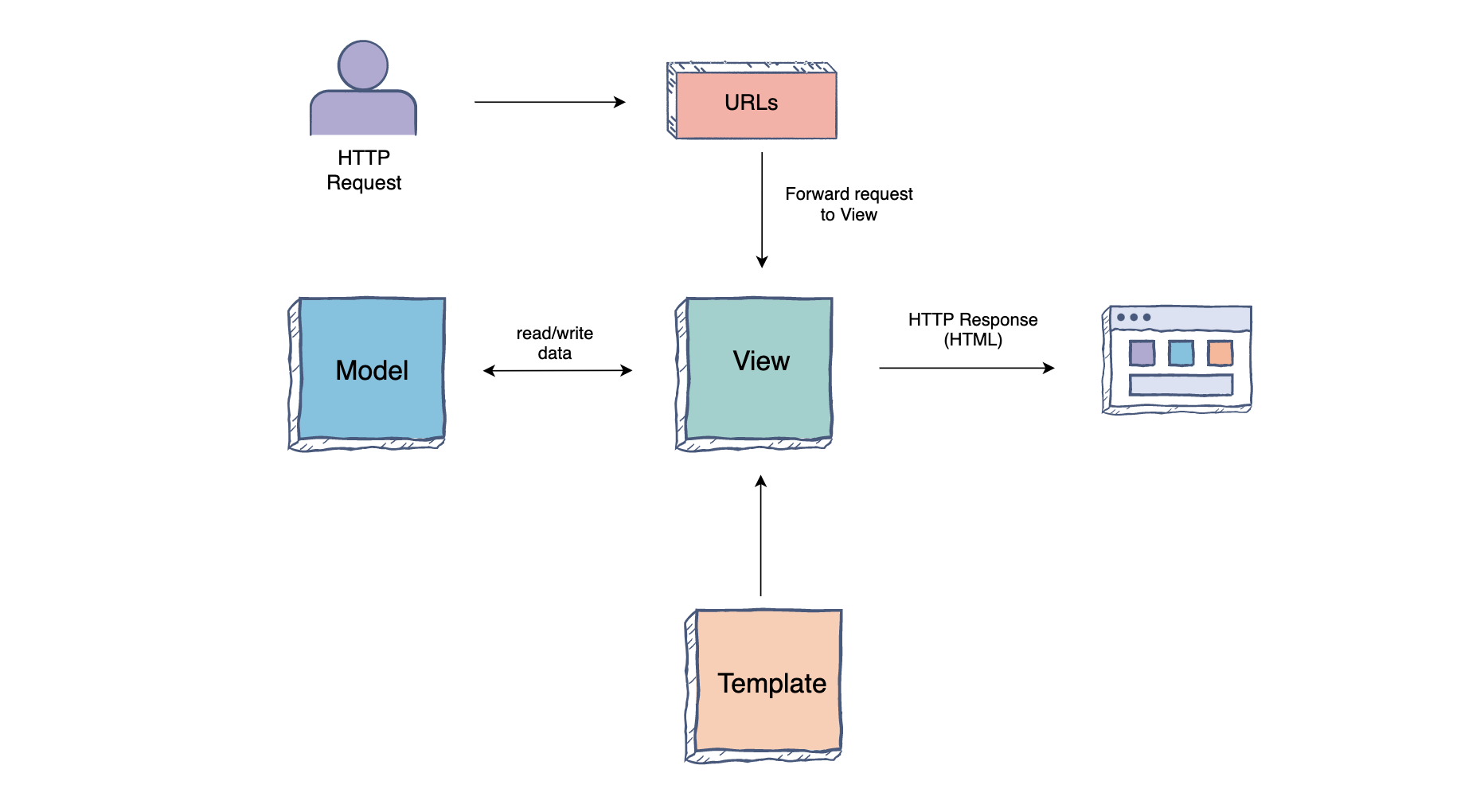
- Model: defines the structure of the database
- View: defines the logic that returns something from an HTTP request.
- Template: defines the structure of how a web page will look with plaintext information that can be read by someone who doesn’t necessarily program
These constituent parts are put in their own distinct files named for their purpose in Django web applications. In addition, you might have a URL mapper that deals with routing to a specific view for different endpoints.